website-breaker
v1.0.3
Published
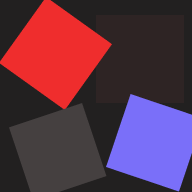
Downloads
16
Readme
Website -breaker | linkedin
Purpose
Website breaker allows you to simulate a breaking effect on your webpages.
Examples
An example usage on my personal portfolio.
An example usage on amazon's landing page.
Here is a code sandbox in react for a more interactive experience.
Installation
With node and npm installed, you can run:
npm i website-breaker
Usage
Using website is very simple;
Vanilla js
// index.js
import breakWebPage from "website-breaker";
document.getElementById("dangerous-button")
.addEventListener("click", () => breakWebPage());
// index.html
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="button.css">
<script src="index.js"></script>
</head>
<body>
<button id="dangerous-button"></button>
</body>
</html>
React
The following button will break the webpage-apart whenever its clicked.
import breakWebPage from "website-breaker";
export const BreakButton = () => {
return (
<button
id="break-website-button"
class="btn"
// destroys the webpage wen clicked
onClick={() => breakWebPage()}
>
Break Me
</button>
);
};
Vue
<template>
<div class="button" @click="destroy" >
Destroy the web-page
</div>
</template>
<script lang="ts">
import { defineComponent } from "vue";
import breakWebPage from "website-breaker";
export default defineComponent({
name: "page-destroyer",
props: {
msg: String,
},
methods: {
destroy(){
breakWebPage();
}
}
});
</script>
Others
While we only presented examples for vue and react, website-breaker is framework independent and would likely work with all of them.