ts-graphql-client
v1.1.7
Published
Typed client GraphQL using Apollo
Downloads
74
Maintainers
Readme
Typescript client GraphQL 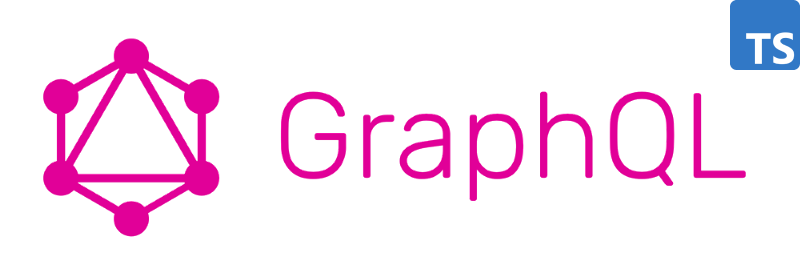
This client is an abstraction of Apollo client to be used with typed methods.
Features
- Create a client
- Call mutation GraphQL
- Call query GraphQL
- Stringify a query or mutation without use client
Installation
This is a Node.js module.
Before installing, download and install Node.js. Node.js 14.0 or higher is required.
npm install ts-graphql-client
or
yarn add ts-graphql-client
How to use
import { GraphQLClient } from 'ts-graphql-client'
type User = {
id: string;
name: string;
username: string;
email: string;
phone: string;
}
const client = new GraphQLClient('https://api.graphqlplaceholder.com/')
async function main() {
const users = await client
.query<{ data: User[] }, {}>(
'users',
{},
{
data: {
id: true,
name: true,
username: true,
email: true,
phone: true,
},
}
)
.then((res) => res.toJSON().data)
console.log(users)
}
main()
You can test it with stackblitz : ts-graphql-client-example
Details
Constructor
Quick start
constructor(uri?: string, verbose?: boolean);
new GraphQLClient('http://localhost:3000/graphql');
With options
constructor(options?: ClientOptions, verbose?: boolean);
new GraphQLClient({
uri: 'http://localhost:3000/graphql',
fetch: fetch as any,
});
More information about available options, read apollo-boost
Queries
query<ResultType, SearchType>(name: string, parameters: ClientParameters<SearchType>, attribute: ClientAttribute<UnArray<ResultType>>, callback: (data: ClientResult<ResultType>, err: ClientError) => void): void;
query<ResultType, SearchType>(name: string, parameters: ClientParameters<SearchType>, attribute: ClientAttribute<UnArray<ResultType>>): Promise<ClientResult<ResultType>>;
mutate<ResultType, SearchType>(name: string, parameters: ClientParameters<SearchType>, attribute: ClientAttribute<UnArray<ResultType>>, callback: (data: ClientResult<ResultType>, err: ClientError) => void): void;
mutate<ResultType, SearchType>(name: string, parameters: ClientParameters<SearchType>, attribute: ClientAttribute<UnArray<ResultType>>): Promise<ClientResult<ResultType>>;
Note
You can use queryToString
to parse your typed query without use client
Hint
To generate types, we suggest to install graphql-code-generator
License
Property of traveljuice