testgear-adapter-jest
v1.1.0
Published
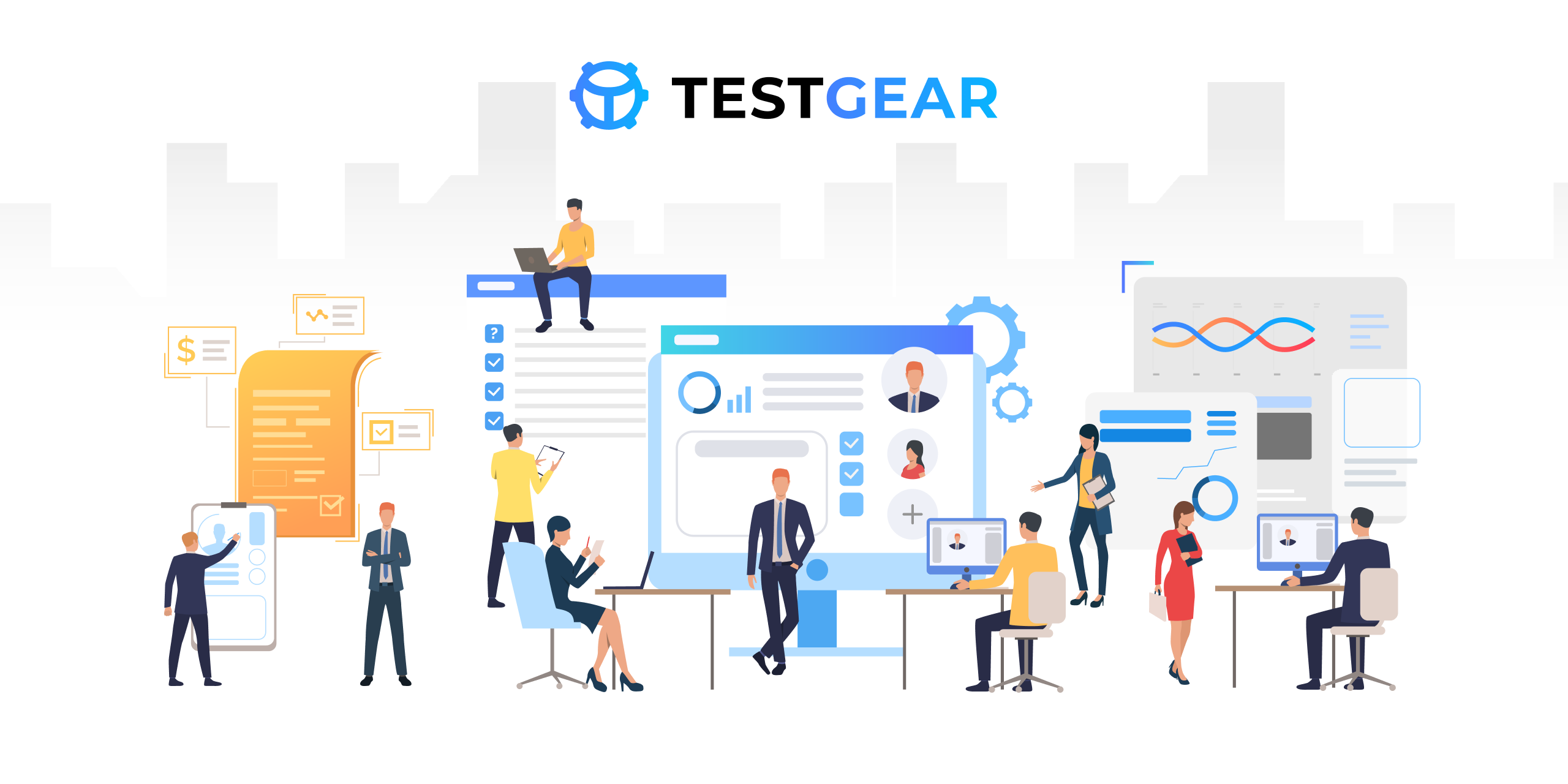
Downloads
1
Readme
TestGear TMS adapters for Jest
Getting Started
Compatibility
| TestGear | Adapter | |----------|---------| | 3.5 | 1.0 | | 4.0 | 1.1 |
Installation
npm install testgear-adapter-jest
Usage
API client
To use adapter you need to install testgear-api-client
:
npm install testgear-api-client
Configuration
File
- You need to set custom jest test environment, setup and teardown in
jest.config.js
.
module.exports = {
testEnvironment: 'testgear-adapter-jest',
globalSetup: 'testgear-adapter-jest/dist/globalSetup.js',
globalTeardown: 'testgear-adapter-jest/dist/globalTeardown.js',
testEnvironmentOptions: {
url: '<url>',
privateToken: '<token>',
projectId: '<id>',
configurationId: '<id>',
testRunId: '<optional id>',
},
};
- You also can extract environment configuration to external config and launch tests with
jest --config ./testgear.jest.config.js
.
// testgear.jest.config.js
const defaultConfig = require('./jest.config');
module.exports = {
...defaultConfig,
testEnvironment: 'testgear-adapter-jest',
globalSetup: 'testgear-adapter-jest/dist/globalSetup.js',
globalTeardown: 'testgear-adapter-jest/dist/globalTeardown.js',
testEnvironmentOptions: {
url: '<url>',
privateToken: '<token>',
projectId: '<id>',
configurationId: '<id>',
testRunId: '<optional id>',
adapterMode: <optional>,
automaticCreationTestCases: <optional boolean>
},
};
- Fill parameters with your configuration, where:
url
- location of the TMS instanceprivateToken
- API secret key- go to the https://{DOMAIN}/user-profile profile
- copy the API secret key
projectId
- ID of project in TMS instance.- create a project
- open DevTools -> network
- go to the project https://{DOMAIN}/projects/{PROJECT_ID}/tests
- GET-request project, Preview tab, copy id field
configurationId
- ID of configuration in TMS instance.- create a project
- open DevTools -> network
- go to the project https://{DOMAIN}/projects/{PROJECT_ID}/tests
- GET-request configurations, Preview tab, copy id field
testRunId
- id of the created test run in TMS instance.testRunId
is optional. If it is not provided, it is created automatically.testRunName
- parameter for specifying the name of test run in TMS instance.testRunName
is optional. If it is not provided, it is created automatically.adapterMode
- adapter mode. Default value - 0. The adapter supports following modes:- 0 - in this mode, the adapter filters tests by test run ID and configuration ID, and sends the results to the test run.
- 1 - in this mode, the adapter sends all results to the test run without filtering.
- 2 - in this mode, the adapter creates a new test run and sends results to the new test run.
automaticCreationTestCases
- mode of automatic creation test cases. Default value - false. The adapter supports following modes:- true - in this mode, the adapter will create a test case linked to the created autotest (not to the updated autotest).
- false - in this mode, the adapter will not create a test case.
Command line
You can also specify options via cli arguments jest --testEnvironment testgear-adapter-jest --testEnvironmentOptions "{\"url\":\"<url>\",\"privateToken\":\"<token>\",\"projectId\":\"<id>\",\"configurationId\":\"<id>\",\"testRunId\":\"<optional id>\",\"automaticCreationTestCases\": <optional boolean>}" --globalSetup testgear-adapter-jest/dist/globalSetup.js --globalTeardown testgear-adapter-jest/dist/globalTeardown.js
Methods
Methods can be used to specify information about autotest.
Description of metadata methods:
testgear.workItemIds
- linking an autotest to a test casetestgear.displayName
- name of the autotest in the TMS system (can be replaced with documentation strings)testgear.externalId
- ID of the autotest within the project in the TMS Systemtestgear.title
- title in the autotest cardtestgear.description
- description in the autotest cardtestgear.labels
- tags in the work itemtestgear.link
- links in the autotest card
Description of methods:
testgear.addLinks
- links in the autotest resulttestgear.addAttachments
- uploading files in the autotest resulttestgear.addMessage
- information about autotest in the autotest result
Examples
Simple test
test('All annotations', () => {
testgear.externalId('all_annotations');
testgear.displayName('All annotations');
testgear.title('All annotations title');
testgear.description('Test with all annotations');
testgear.labels(['label1', 'label2']);
testgear.addMessage('This is a message');
testgear.addLinks([
{
url: 'https://www.google.com',
title: 'Google',
description: 'This is a link to Google',
type: 'Related',
},
]);
testgear.addAttachments([join(__dirname, 'attachment1.txt')]);
testgear.addAttachments('This is a custom attachment', 'custom.txt');
expect(1).toBe(1);
});
Parameterized test
test.each([1, 2, 3, 4])('Primitive params', (number) => {
testgear.params(number);
expect(number).toBe(number);
});
test.each([
{
a: 1,
b: 2,
sum: 3,
},
{
a: 2,
b: 3,
sum: 5,
},
{
a: 4,
b: 3,
sum: 5,
}
])('Object params', (params) => {
testgear.params(params);
expect(params.a + params.b).toBe(params.sum);
});
Contributing
You can help to develop the project. Any contributions are greatly appreciated.
- If you have suggestions for adding or removing projects, feel free to open an issue to discuss it, or directly create a pull request after you edit the README.md file with necessary changes.
- Please make sure you check your spelling and grammar.
- Create individual PR for each suggestion.
- Please also read through the Code Of Conduct before posting your first idea as well.
License
Distributed under the Apache-2.0 License. See LICENSE for more information.