react-img-grid
v1.0.4
Published
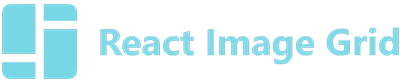
Downloads
5
Readme
A responsive staggered image-grid react component with lightbox and lazy-load support.
Install
npm install --save-dev react-image-grid
Basic Usage
import ImageGrid from 'react-image-grid';
function App(props){
const IMAGES = [
{
src: 'https://example.com/my-image-1.jpg',
alt: 'Sample Alt Text 1',
},
{
src: 'https://example.com/my-image-2.jpg',
alt: 'Sample Alt Text 2',
},
];
return (
<ImageGrid images={IMAGES}/>
);
}
Lazy Loading Images
Lazy loading images can be achieved using the onBottomVisible prop which takes a callback that is called when the bottom of the image grid comes into view.
Here's an example of how you might setup lazy loading in an app:
import React, {useState} from 'react';
import ImageGrid from 'react-image-grid';
function App(props){
const [imgs, setImgs] = useState([]);
async function loadMoreImages(){
try{
let page_num = imgs / [Per Page Image Count];
await fetch(`https://[Your Image Endpoint]/${page_num}`);
setImgs([...imgs, new_imgs]);
}catch(err)
console.error(err);
}
}
return (
<ImageGrid
images={imgs}
onBottomVisible={loadMoreImages}
/>
);
}
Props
| Name| Type | Description | |--|--|--| | images | array | Array of objects with any valid combination of properties described in the Image Properties section below. | | colThreshold | integer | Number of pixels at which to add a column. Number of columns will be [parent element width] / colThreshold. minColThreshold will override this if the calculated column count is lower than the minimum. | | minColCount | integer| The minimum number of columns to use when column count is being calculated. Not applicable when absoluteColCount is set.| | absoluteColCount | integer | An absolute number of columns to display. Overrides dynamically determining column count via minColCount and colThreshold. | | lightboxEnabled | boolean | Whether or not to show an image in a pop-up lightbox when the image is clicked. Defaults to true. | onBottomVisible | callback | Called when the bottom of the grid is visible. Supply a function that loads more images to dynamically add images as the user scrolls down the page.| | bottomTriggerThreshold| integer| The number of pixels before the bottom of the image grid is actually seen to call the onBottomVisible callback. Defaults to 350. | | onImageClick| callback | Called when an image in the grid is clicked. First parameter is the index of the image in the grid. Second parameter is the image object. |
Image Properties
|Property Name| Type | Description |
|--|--|--|
| src | string | The url pointing to the image. (Required)|
| alt | string | Alt text for the image (Required)|
| title | string | Text to be displayed on image hover if hoverTitle
is enabled. (Optional) |
| showHoverTitle | boolean | Whether or not to display the title
on mouse hover. (Optional. Defaults to true.) |
| props | object | Props directly passed to the underlying image elements themselves. |