pathre
v1.0.0
Published
[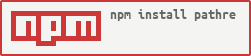](https://nodei.co/npm/pathre/)
Downloads
5
Maintainers
Readme
path-resolver utility functions for node
Pathre
consists of validation and get functions which make dealing with path super simple and easy :ok_hand:
Usage
Validation functions
Get functions
Other
Getting Started
clone the repo:
git clone [email protected]:jimmy02020/pathre.git
cd pathre
Using npm:
$ npm install pathre
Validation:
const { check } = require('pathre')
isPathValid(path)
Validate given path. If path is valid returns true, otherwise returns false.
Example
check.isPathValid('whatever') // false
check.isPathValid(path.join(__dirname)) // true
isPathDir(path)
If path is for directory (not file) returns true, otherwise returns false.
Example
check.isPathDir('/path/here/') // true
check.isPathDir('/path/here/test.txt') // false
isPathFile(path)
If path is for file (not directory) returns true, otherwise returns false.
Example
check.isPathFile('/path/here/test.txt') // true
isExist(path, callback)
If path is valid/existent returns true, otherwise returns false.
NOTE: Path can be valid path but not for existence path. If you check for existence use isExist
if you validate the path before create it use isValid
.
Example
isExist('/here/dir', (isExsist) => {
if(isExsist){
// do something
console.log("valid");
} else {
// do something else
console.log("invalid");
}
})
isFileZeroSize(path, callback)
If file is valid and zero size then true, otherwise returns false.
Example
isFileZeroSize('/here/dir/empty.txt', (isZero) => {
if(isZero){
// do something
console.log("file is empty");
} else {
// do something else
console.log("file has info in it empty");
}
})
Get functions:
const { get } = require('pathre')
directory(path)
Returns string has directory name, whether the path is with file name or without it.
Example
get.directory('/path/here/.env.test') // '/path/here
fileName(path)
Returns string has file name.
Example
get.fileName('/path/here/.env.test') // .env.test
fileExt(path)
Returns string has file extension.
Example
get.fileExt('/path/here/.env.test') // env
get.fileExt('/path/here/filename.txt') // txt
fileStat(path, callback)
Gets text statistics from file. The function is implementation of textics-stream
The callback gets two arguments (err, stat)
.
stat
object consists of:
lines
words
chars
spaces
Example
get.fileStat('dir/dir2/file.txt', (err, stat) => {
console.log(stat);
//
{
lines: 1830,
words: 4483,
chars: 12584,
spaces: 3004,
}
//
});
pathType(path)
Gets path type, file or directory.
returns {isDir, isFile}
.
Example
const type = get.pathType('dir/here/emptyfile.txt')
console.log(type);
//
{
isDir: false,
isFile: true,
}
//
pathStat(path, callback)
Gets the main properties from path.
The callback gets two arguments (err, stat)
.
stat
object consists of:
isValid
isDir
isFile
size
Example
get.pathStat('dir/here/emptyfile.txt', (err, stat) => {
console.log(stat);
//
{
isValid: true,
isDir: false,
isFile: true,
size: 0,
}
//
});
Tests
$ npm test
License
This project is licensed under the MIT License