graphql-loopback-subscriptions
v2.0.1
Published
[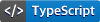](https://github.com/ellerbrock/typescript-badges/) [](https://github.com/ellerbrock/open-source-ba
Downloads
11
Maintainers
Readme
Graphql Loopback Subscription PubSub
This package implements the PubSubEngine Interface from the graphql-subscriptions package and also the new AsyncIterator interface. It allows you to subscription in Loopback graphql-js.
Using as AsyncIterator
Define your GraphQL schema with a Subscription
type:
schema {
query: Query
mutation: Mutation
subscription: Subscription
}
type Subscription {
somethingChanged: Result
}
type Result {
id: String
}
Now, let's create a simple LoopbackPubSub
instance:
import { LoopbackPubSub } from 'graphql-loopback-subscriptions';
const pubsub = new LoopbackPubSub();
Now, implement your Subscriptions type resolver, using the pubsub.asyncIterator
to map the event you need:
const SOMETHING_CHANGED_TOPIC = 'something_changed';
export const resolvers = {
Subscription: {
somethingChanged: {
subscribe: () => pubsub.asyncIterator(SOMETHING_CHANGED_TOPIC),
},
},
}
Subscriptions resolvers are not a function, but an object with
subscribe
method, that returnsAsyncIterable
.
Dynamically create a topic based on subscription args passed on the query:
export const resolvers = {
Subscription: {
somethingChanged: {
subscribe: (_, args) => pubsub.asyncIterator(`${SOMETHING_CHANGED_TOPIC}.${args.relevantId}`),
},
},
}
Using both arguments and payload to filter events
import { withFilter } from 'graphql-subscriptions';
export const resolvers = {
Subscription: {
somethingChanged: {
subscribe: withFilter(
() => loopbackPubSub.asyncIterator('trigerName'),
(payload, variables, arg0, arg1) => {
try {
loopbackPubSub.subscribe('trigger-name', null, variables);
} catch (ex) {
console.log(ex);
}
return true;
},
),
},
},
}