dibujo
v5.0.22
Published
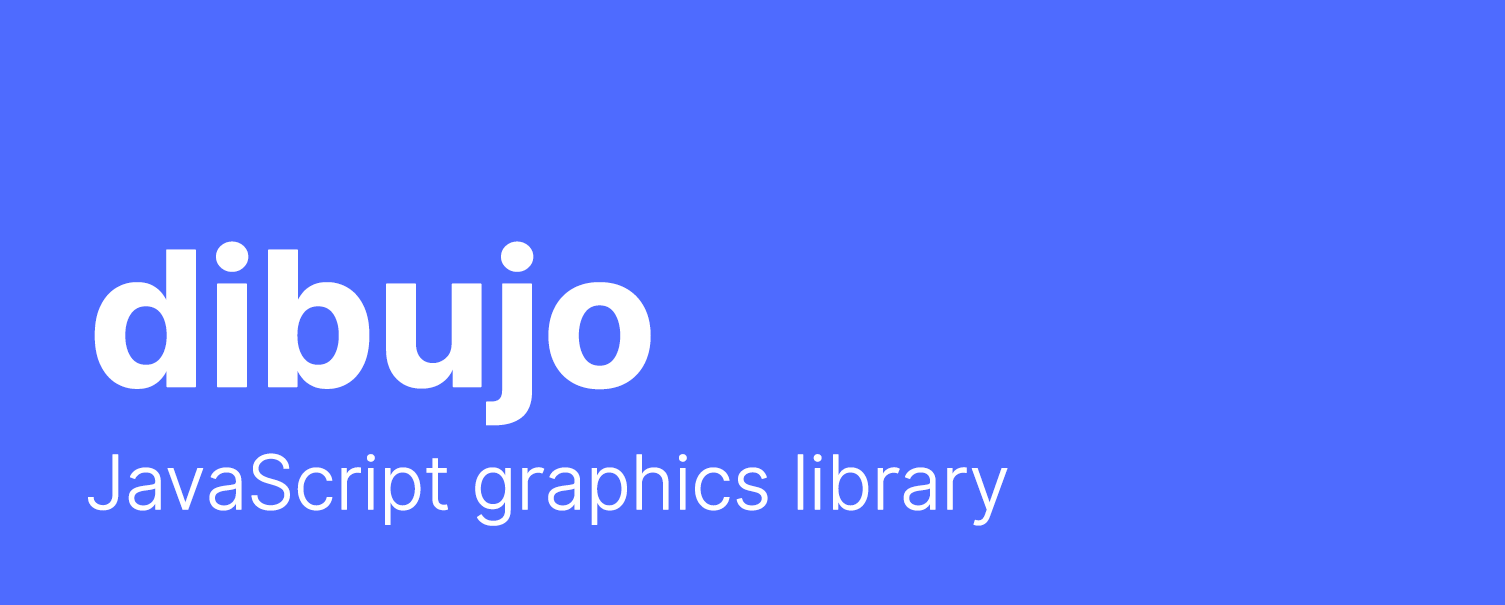
Downloads
11
Readme
Instalation
How to use it
const render = new dibujo.Render()
Scene
const scene = new dibujo.Scene('#FF00FF')
const rect = new dibujo.Rect({
position: new dibujo.Vector(10, 10),
width: 100,
height: 100,
color: 'blue'
})
scene.add(rect)
render.setScene(scene)
Graphics
- Rect
- Circle
- Picture
- Line
- Poligon
- Text
- Arc
Picture
const picture = new dibujo.Picture({
position: new dibujo.Vector(10, 10),
width: 100,
height: 100,
src: './apple.png'
})
| Param | Type | Description | | --- | --- | --- | | position | object | This object contains the position of the image | | src | string | This will be the location where the image is saved | | width | number | The width | | opacity | number | The opacity | | height | number | The height |
Rect
const rect = new dibujo.Rect({
position: new dibujo.Vector(10, 10),
width: 100,
height: 100,
color: 'blue'
})
| Param | Type | Description | | --- | --- | --- | | position | object | This object contains the position of the rect | | color | string | The color of the rect | | width | number | The width | | height | number | The height | | stroke | boolean | Draw stroke | | lineColor | number | Stroke color | | lineWidth | number | Line width |
Text
const text = new dibujo.Text({
position: new dibujo.Vector(10, 10),
content: 'Hello World'
})
| Param | Type | Description | | --- | --- | --- | | position | Point | The coordinates of the text | | content | string | The content | | style | object | This is the style of the text |
Line
const line = new dibujo.Line({
start: new dibujo.Vector(10, 10),
end: new dibujo.Vector(20, 20),
color: 'blue'
})
| Param | Type | Description | | --- | --- | --- | | start | Point | The start coordinate of the line | | end | Point | The end coordinate of the line | | color | string | This is the color of the line |
Poligon
const poli = new dibujo.Poligon({
cords: [
{x: 10, y: 10},
{x: 20, y: 20},
{x: 10, y: 20}
],
color: 'blue'
})
| Param | Type | Description | | --- | --- | --- | | cords | Array | Array of the vertex of the poligon | | fill | boolean | Fill the poligon | | stroke | boolean | Stroke the poligon | | strokeColor | string | This is the color of the lines of the poligon | | color | string | This is the color of the poligon |
Circle
const circle = new dibujo.Circle({
position: new dibujo.Vector(10, 10),
radius: 10,
color: 'blue'
})
| Param | Type | Description | | --- | --- | --- | | position | Point | Position of the circle | | radius | number | Radius of the circle | | color | string | Color of the circle | | stroke | boolean | Show stroke | | lineWidth | number | Width of the line | | lineColor | string | Color of the line |
Arc
const arc = new dibujo.Arc({
position: new dibujo.Vector(10, 10),
radius: 10,
color: 'blue'
})
| Param | Type | Description | | --- | --- | --- | | color | string | Color of the arc | | position | Point | Position of the arc | | radius | number | Radius of the arc | | lineWidth | number | Width of the line | | stroke | boolean | Show stroke | | lineColor | string | Color of the line | | eAngl | number | End angle of the arc | | aAngl | number | Start angle of the arc |