dessert-showdown
v1.0.3
Published
[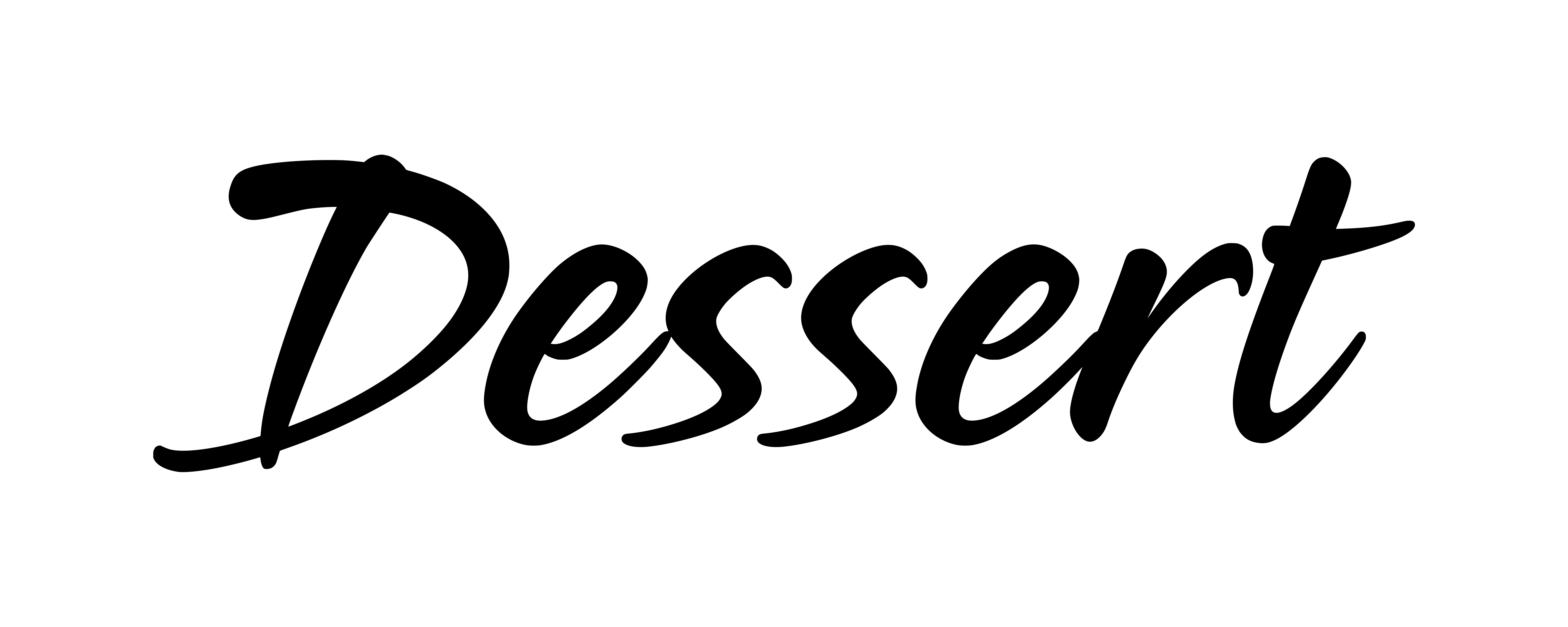](https://dessert.dev/)
Downloads
1
Readme
Dessert Showdown
showdown, but implemented with Rust and WebAssembly
Table of contents
Usage
var showdown = require('showdown'),
converter = new showdown.Converter(),
text = '# hello, markdown!',
html = converter.makeHtml(text);
Output
<h1 id="hellomarkdown">hello, markdown!</h1>
API
Options
You can change some of showdown's default behavior through options.
Setting options
Options can be set:
Globally
Setting a "global" option affects all instances of showdown
showdown.setOption('optionKey', 'value');
Locally
Setting a "local" option only affects the specified Converter object. Local options can be set:
through the constructor
var converter = new showdown.Converter({optionKey: 'value'});
through the setOption() method
var converter = new showdown.Converter(); converter.setOption('optionKey', 'value');
Getting an option
Showdown provides 2 methods (both local and global) to retrieve previous set options.
getOption()
// Global
var myOption = showdown.getOption('optionKey');
//Local
var myOption = converter.getOption('optionKey');
getOptions()
// Global
var showdownGlobalOptions = showdown.getOptions();
//Local
var thisConverterSpecificOptions = converter.getOptions();
Retrieve the default options
You can get showdown's default options with:
var defaultOptions = showdown.getDefaultOptions();
Valid Options
noHeaderId: (boolean) [default false] Disable the automatic generation of header ids. Setting to true overrides prefixHeaderId
customizedHeaderId: (boolean) [default false] Use text in curly braces as header id. Example:
## Sample header {real-id} will use real-id as id
ghCompatibleHeaderId: (boolean) [default false] Generate header ids compatible with github style (spaces are replaced with dashes and a bunch of non alphanumeric chars are removed)
prefixHeaderId: (string/boolean) [default false] Add a prefix to the generated header ids. Passing a string will prefix that string to the header id. Setting to
true
will add a generic 'section' prefix.rawPrefixHeaderId: (boolean) [default false] Setting this option to true will prevent showdown from modifying the prefix. This might result in malformed IDs (if, for instance, the " char is used in the prefix). Has no effect if prefixHeaderId is set to false.
rawHeaderId: (boolean) [default false] Remove only spaces, ' and " from generated header ids (including prefixes), replacing them with dashes (-). WARNING: This might result in malformed ids
headerLevelStart: (integer) [default 1] Set the header starting level. For instance, setting this to 3 means that
# foo
will be parsed as
<h3>foo</h3>
~~literalMidWordAsterisks: (boolean) [default false] Turning this on will stop showdown from interpreting asterisks in the middle of words as
<em>
and<strong>
and instead treat them as literal asterisks.~~strikethrough: (boolean) [default false] Enable support for strikethrough syntax.
~~strikethrough~~
as<del>strikethrough</del>
tables: (boolean) [default false] Enable support for tables syntax. Example:
| h1 | h2 | h3 | |:------|:-------:|--------:| | 100 | [a][1] | ![b][2] | | *foo* | **bar** | ~~baz~~ |
See the wiki for more info
tasklists: (boolean) [default false] Enable support for GFM tasklists. Example:
- [x] This task is done - [ ] This is still pending
simpleLineBreaks: (boolean) [default false] Parses line breaks as
<br>
, without needing 2 spaces at the end of the linea line wrapped in two
turns into:
<p>a line<br> wrapped in two</p>
requireSpaceBeforeHeadingText: (boolean) [default false] Makes adding a space between
#
and the header text mandatoryghMentions: (boolean) [default false] Enables github @mentions, which link to the username mentioned
ghMentionsLink: (string) [default
https://github.com/{u}
] Changes the link generated by @mentions. Showdown will replace{u}
with the username. Only applies if ghMentions option is enabled. Example:@tivie
with ghMentionsOption set to//mysite.com/{u}/profile
will result in<a href="//mysite.com/tivie/profile">@tivie</a>
emoji: (boolean) [default false] Enable emoji support. Ex:
this is a :smile: emoji
For more info on available emojis, see https://github.com/showdownjs/showdown/wiki/Emojis
Installation
With npm:
npm install dessert-showdown
License
This software is licensed under the MIT license (see LICENSE).
Contributing
See CONTRIBUTING.md