@toreda/fate
v0.7.0
Published
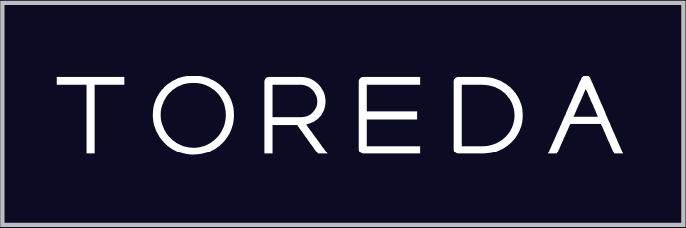
Downloads
16
Readme
@toreda/fate
Return function results, errors, status, and logs with one object.
Install
With yarn (preferred):
yarn add @toreda/fate
With NPM:
npm install @toreda/fate
Usage
Library Usage
Typescript
import {Fate} from '@toreda/fate';
function request(): Fate<string> {
// Can set the type of payload by calling Fate with a type arg.
// Payload type defaults to unknown.
const fate = new Fate<string>();
const result = someAction();
if (thereIsAProblem()) {
return fate.error('whatever is passed will get converted to an Error obj if it isnt one');
}
fate.state.payload = result;
}
const fate = request();
if (fate.isFailure()) {
// fate.state.errorLog holds all the errors that were attached to the fate
}
if (fate.isSuccess()) {
// fate.state.payload
}
// Returns Error[] if fate fails, the payload if fate succeeds, or Error[] with a single error if payload is null/undefined
console.log(fate.getData());
// Converts all the state data into a string
const serialized = fate.serialize();
// Uses serialized state data to rebuild a fate
const fromSerial = new Fate({serialized});
TypeScript
import {Fate} from '@toreda/fate';
const fate = new Fate<never>();
if (fate.success()) {
console.info(`Success`);
} else {
console.info(`Failed`);
}
import {Fate} from '@toreda/fate';
function isPositive(value: number): Fate<boolean> {
// Fate instance containing a boolean with an initial value `false`.
const fate = new Fate<boolean>({
data: false
});
// Sets success to false & sets an error code.
if (typeof value !== 'number') {
return fate.setErrorCode('non_number_value');
}
fate.data = value >= 0;
return fate.setSuccess(true);
}
const result = isPositive(1);
if (result.success()) {
// Function succeeded.
if (fate.data === true) {
console.info(`${value} is positive`);
} else {
console.info(`${value} is negative`);
}
} else {
console.error(`isPositive failed with error code: ${result.errorCode()});
}
Build
Build (or rebuild) the config package:
With Yarn (preferred):
yarn install
yarn build
With NPM:
npm install
npm run-script build
Testing
Config implements unit tests using jest. Run the following commands from the directory where config has been installed.
With yarn (preferred):
yarn install
yarn test
With NPM:
npm install
npm run-script test
Legal
License
MIT © Toreda, Inc.
Copyright
Copyright © 2019 - 2022 Toreda, Inc. All Rights Reserved.
https://www.toreda.com