@stash-it/dev-tools
v0.0.3
Published
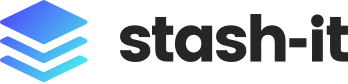
Downloads
304
Maintainers
Readme
@stash-it/dev-tools
Set of tools for development purposes.
If you want to create an adapter or a plugin for stash-it, you can use this package to help you with that.
Installation
npm
npm install @stash-it/dev-tools
deno
deno add @stash-it/dev-tools
yarn
yarn dlx jsr add @stash-it/dev-tools
pnpm
pnpm dlx jsr add @stash-it/dev-tools
bun
bunx jsr add @stash-it/dev-tools
Usage
runAdapterTests
If you want to create an adapter, it should pass all of those tests. Here's how you can use them.
Oh, and you should use vitest
for testing, the function uses it under the hood.
If you're not using vitest
yet, you should start using it. It's awesome.
Perhaps in the future, I'll add support for other testing libraries or be more abstract, but for now it is what it is.
// Vitest stuff
import { describe } from "vitest";
import { runAdapterTests } from '@stash-it/dev-tools';
// Your adapter.
import { YourAdapter } from './your-adapter';
describe('YourAdapter', () => {
const adapter = new YourAdapter();
// Add whatever validation or other stuff you need.
// Run the tests.
runAdapterTests(adapter);
});
This helper function accepts an adapter instance and runs tests on it. It will throw an error if any of the tests fail. It is also capable of cleaning up after self. Meaning, if you're operating on a persistent storage, it will delete all the items created during the tests.
getHandler
If you want to creat a plugin, you can use this helper function to get a handler for a specific hook. Here's how you can use it:
// Vitest stuff
import { describe, it, expect } from "vitest";
import { getHandler } from '@stash-it/dev-tools';
// Your plugin.
import { createPlugin } from './your-plugin';
describe('YourPlugin', () => {
it('does something on buildKey', async () => {
const plugin = createPlugin();
const key = "key";
const handler = getHandler('buildKey', plugin);
// Here is where you can test the handler that you created.
const result = await handler(key);
// expect ... etc.
});
});
Why not simply do this?
const handler = plugin.hookHandlers.buildKey;
Well ... current types set around plugins are not that great. This helper function will throw an error if the handler is not a function. Also, thanks to the argument passed (hook name), it will know what arguments the handler should accept etc. And you don't need to reach to plugin internals. You simply request a handler for a specific hook and that's it.
License
MIT
Contribution
Feel free to open an issue or a pull request.